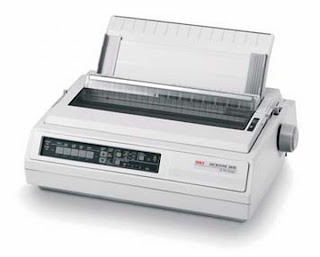
In web based applications, printing is a common requirement.User can print a web page by using the printing utilities of the browser.This may leave users at a surprised state because most of the time the format of the printed web page is messed up.For this reason a "Printer friendly" version of the page is provided. There are many ways to create a printer friendly version using server-side scripting. A separate request for printer friendly page is sent to the server and the server responses with a well formatted printable page. While these ways are completely workable, an elegant and client based approach is the use of only CSS to provide a printable version.
To accomplish this, we need to follow the next simple processes.
1. Create a seperate CSS file that controls the appearence of the page's elements for printable version.Let's call it "printable.css".
2. Include the CSS into the page that will be printed with the following syntax.
<link rel="stylesheet" href="--CSS folder path--" media="print" />
The important part here is the "media" attribute. When the value of this attribute is set to "print", the browser automatically uses this CSS file to format the page while printing.
Let's see a quick example. The layout of our page could be the following:
<html>
<head>
<link rel="stylesheet" href="<CSS folder path>/printable.css" type="text/css" media="print" />
</head>
<body>
<div id="header">
<!- HTML elements for header(logo,banner) -->
</div>
<div id="top_menu">
<!- HTML elements for top menu goes here -->
</div>
<div id="main_content">
<!- HTML elements for page content goes here -->
</div>
<div id="footer">
<!- HTML elements for footer goes here -->
</div>
</body>
</html>
While printing this page, we would probably want to print only the main content and escape the printing of the header, menu or footer. We can accomplish this by using the "printable.css" as follows:
#header,#top_menu, #footer {display:none}
We can add a link or button to anywhere in the page above to provide printing functionality.
<a href="#" onclick="window.print(); return false"> Print </a>
When user clicks on the "Print" link, the browser uses the "printable.css" and hides all portions except the content of the "main_content" element while printing.The result is a formatted printer friendly version of the page.We can fine tune the "printable.css" to take more control over the printable version.